Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | ||||||
2 | 3 | 4 | 5 | 6 | 7 | 8 |
9 | 10 | 11 | 12 | 13 | 14 | 15 |
16 | 17 | 18 | 19 | 20 | 21 | 22 |
23 | 24 | 25 | 26 | 27 | 28 | 29 |
30 | 31 |
Tags
- css
- 영속성 컨텍스트
- 노마드코더
- html
- javascript
- TODO
- frontend
- ORM
- 단방향
- web
- JPA
- 바닐라js
- java
- SBERT
- 다대다
- Django
- 장고
- 트랜잭션
- nomadcoder
- AWS
- 매핑
- React
- JS
- 일대다
- 장고독학
- python
- 플러시
- clonecoding
- 프론트엔드
- 다대일
Archives
- Today
- Total
꿈꾸는 새벽하늘
[자료구조] C언어로 스택(Stack) 구현하기 본문
Stack ADT
push(item) : 스택이 full이 아닐 때 item을 스택에 삽입
pop() : 스택이 empty가 아닐 때 top의 item을 반환 후 제거
peek() : 스택이 empty가 아닐 때 top의 item을 반환 (*제거하지 않음)
isFull() : 스택이 포화상태인지 확인
isEmpty() : 스택이 공백상태인지 확인
Source Code
// 배열을 이용한 스택을 전역 변수로 구현하는 방법
#include <stdio.h>
#include <stdlib.h>
#define MAX_STACK_SIZE 100 // 스택의 최대 크기
typedef int element; // 데이터의 자료형
element stack[MAX_STACK_SIZE]; // 1차원 배열
int top = -1;
// 스택이 비어 있는 상태인지 확인하는 함수
int isEmpty() {
return (top == -1);
}
// 스택이 가득 찬 상태인지 확인하는 함수
int isFull() {
return (top == (MAX_STACK_SIZE - 1));
}
// 삽입 함수
void push(element item) {
if (isFull()) {
printf("스택이 가득 찼습니다.\n");
return;
}
else
stack[++top] = item;
}
// 삭제 함수
element pop() {
if (isEmpty()) {
printf("스택이 비어 있습니다.\n");
exit(1); // 에러 발생 시 강제 종료
}
else
return stack[top--];
}
// 피크 함수
element peek() {
if (isEmpty()) {
printf("스택이 비어 있습니다.\n");
exit(1); // 에러 발생 시 강제 종료
}
else
return stack[top];
}
int main(void) {
push(10);
push(7);
push(6);
push(5);
printf("%d\n", pop());
printf("%d\n", peek());
printf("%d\n", pop());
printf("%d\n", pop());
push(3);
printf("%d\n", pop());
return 0;
}
Output
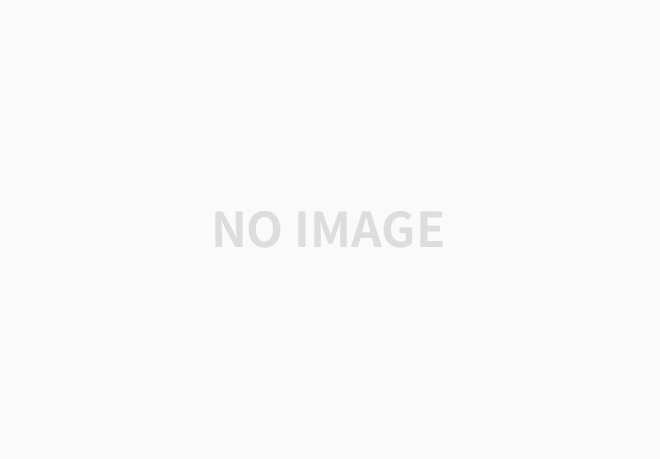
'⭐ Algorithm' 카테고리의 다른 글
[자료구조] 스택(Stack)의 정의 (0) | 2022.01.11 |
---|